Greenshift has several form Element blocks that allow you to build custom forms. Also, Interaction layer feature has form actions, so let’s dive inside.
Currently, form elements have four blocks: Form (it’s working as container), Input (with all types), Textarea, and Label
Form block
First, you need to add a Form block. Then, add an action link where you will process the form. This can be linked to your site or an external site. Keep reading, and we will show examples of form actions for adding posts from the front end.

Form has also option to enable Pretty Styles. This will add some improved styles to all inner input elements. You can also add styles to each input separately or you can use local/global class system
Input block
Options of Input block are totally the same as option of Input HTML tag in browsers
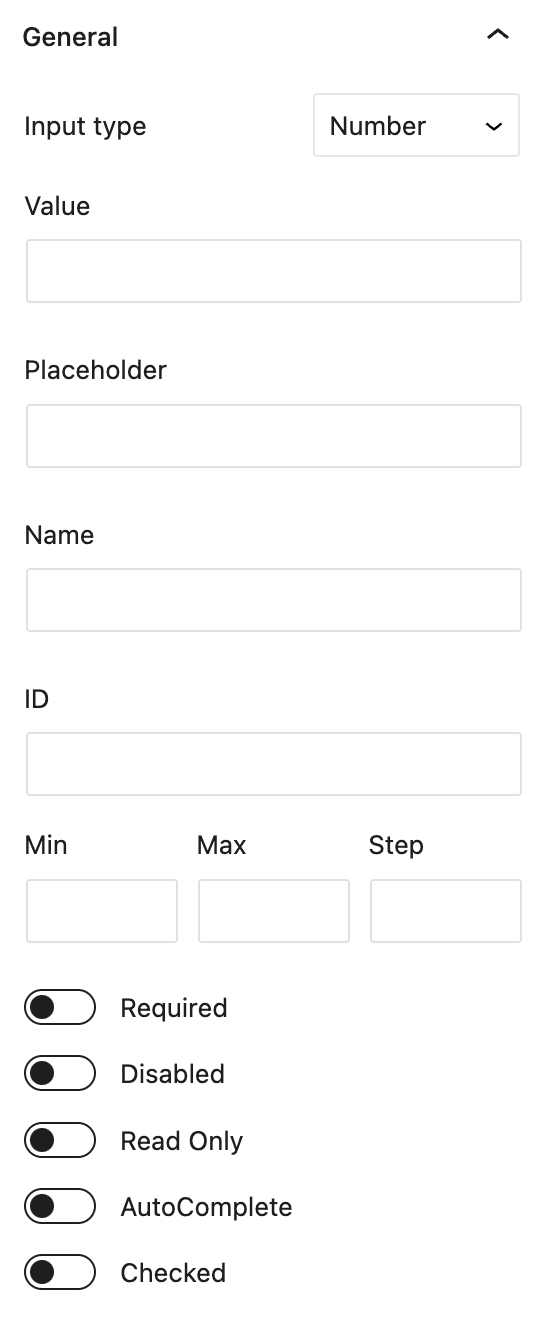
Textarea block
This is the same as input, but it also has Cols/Rows options that control the default size of textarea
Label block
Label block is unnecessary because you can use the placeholder option in inputs. However, placeholders are placed inside the input, and labels are outside. You can also use regular text instead of labels. To make labels to work correctly, you must set “for” option, and it must be equal to ID option of synced input or textarea
Form Interactions
Greenshift has a powerful Interaction Layer feature with specific options for forms.
On change trigger
This trigger works when you change the input value. There is also a special placeholder {{VALUE}} that you can use in actions to get the current value of input after change
This is an example of simple form element interactions with a page.

Here, we enable the Interaction layer on the Input block with Range type. We add the “On input change” trigger, and as an action, we add the setting of a font-size property on an element with the font size change class. As a value, we get the value of input after changing + we add “px” unit to the value
Here is the result of such a block. Use range slider and it will change font size of text element
For more advanced usage, you can also use Rive actions
Action Conditions
In each action, you will find also Condition button, and there are several input conditions by value: less, more then value, between, contains, not contains, checked condition (for checkboxes), equal, not equal)

This is an example of a simple interaction; I added the option to change the background color based on the input value. If the input value is more than specific, I change the background color to red. If it is less, I change it to blue. This can also be used to make conditional inputs that relate to the value of other inputs.
Form Actions
With Interaction layers, you can build interactions that don’t require any server actions. But if you need something to save in the database or send external requests, you must make your action for the form and put a link to this action in the form.
Don’t forget to add input with the type “submit” to the form. This will add trigger action to form
Usually, I use two types of form actions.
GET method for form
When you enable this method, each input value will be available in the resulting URL, and you can access it with $_GET in PHP. For action, you can put the current page link or leave it empty, or put a link on the page where you have a PHP processor
Example. I create a form with input Number and the name “price”.
When you submit such a form, the page’s URL will be site.com/page?price=value, where the value is what the user puts in the field. Now, you can put PHP code on the page
<?php if (!empty($_GET['price'])){
$price = sanitize_text_field($_GET['price']);
//do something with price
}?>
You can use Smart code AI plugin to put PHP on page or use the global function in functions.php of your theme or in custom snippet plugin
POST method for form and admin_post hook
The post method allows for more complex data to be kept in the form because it doesn’t attach query strings to the link. There are many ways to use the post method with WordPress. I want to share a simple example related to one hidden feature of WordPress: the admin_post hook. I discovered it recently, and it’s a very useful hook for making custom form actions.
So, to use the admin_post hook, you must add a link to the admin-post.php file as an action. The final action URL for the form will be
https://site.com/wp-admin/admin-post.php
Next required step is to add special input field to form with hidden type. So, add input block and select it’s type as “hidden”. Put “action” as name and set custom value as value. Example

Keep attention on the value field. Now, you must create a function and put it in the theme’s functions.php or use a custom snippet plugin. Then, put the next code.
add_action( 'admin_post_submit_feature_form', 'submit_feature_form_handler' );
add_action( 'admin_post_nopriv_submit_feature_form', 'submit_feature_form_handler' );
function submit_feature_form_handler() {
}
Pay attention to the action’s name. It’s admin_post + the action name you set for your hidden field. Here, we use two actions, one with the “nopriv” part and one without. The action with the “nopriv” part will be used only if you want to make it work for non-registered users.
It’s important to mention that any kind of user input is dangerous, so you must sanitize it before using, also I recommend making extra security checking, for example, by user capabilities
Here is out full code for making frontend form
add_action( 'admin_post_submit_feature_form', 'submit_feature_form_handler' );
function submit_feature_form_handler() {
// Check if the user is authorized to submit the form
if ( ! current_user_can( 'publish_posts' ) ) {
wp_die( 'Unauthorized user' );
}
// Retrieve form data
$title = !empty($_POST['title']) ? sanitize_text_field( $_POST['title'] ) : '';
$content = !empty($_POST['content']) ? wp_kses_post( $_POST['content'] ) : '';
// Create a new post of type "features"
$post_id = wp_insert_post( array(
'post_title' => $title,
'post_content' => wp_slash($content),
'post_status' => 'publish',
'post_type' => 'request',
) );
// Redirect the user after submission
if ( $post_id ) {
wp_set_object_terms($post_id, 'request_tag', 'new', false);
wp_redirect( get_the_permalink($post_id) );
exit;
} else {
echo 'Unexpected error';
exit;
}
}
This code will use data from our form, it will work only for registered users who have publishing post capabilities. It will create post on your site for Post type Request. And attach tag “new”, then redirect user to this post after publishing.
You can use simple forms not only for post submit but for any purpose, with help of Chat GPT, you can build any form, just ask in prompt to build you function with help of admin_post hook
Example is for simple contact form
// Handle contact form submission
function handle_contact_form() {
// Check if the form is submitted
if (isset($_POST['name']) && isset($_POST['email']) && isset($_POST['message'])) {
$name = sanitize_text_field($_POST['name']);
$email = sanitize_email($_POST['email']);
$message = sanitize_textarea_field($_POST['message']);
// You can perform further validation here if needed
// Example: Send email
$to = '[email protected]';
$subject = 'New Contact Form Submission';
$body = "Name: $name\n\nEmail: $email\n\nMessage:\n$message";
$headers = array('Content-Type: text/html; charset=UTF-8');
// Send email
wp_mail($to, $subject, $body, $headers);
// Redirect back to the page after submission
wp_redirect(get_permalink(), 303);
exit;
} else {
// Redirect to homepage if accessed directly
wp_redirect(home_url());
exit;
}
}
add_action('admin_post_nopriv_handle_contact_form', 'handle_contact_form');
add_action('admin_post_handle_contact_form', 'handle_contact_form');
Of course, it’s better to use it with captcha, chatGPT can help to inject google recaptcha to code